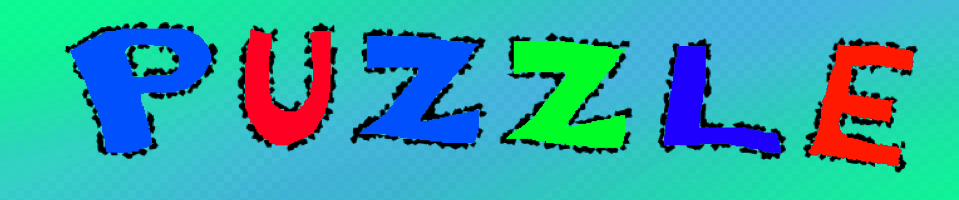
V0.3 Road so far
- Glow puzzle pieces - Chosen, completed, etc..
- Complete Pieces with each other - Grouping
- Gui control for selecting files <- In progress
- Full screen or windowed
- Check image formats
- Save puzzle for later solve
- “Hint” - Where you should put a puzzle piece.
- Special glowing piece which bring more points.
- Prepackaged images
V0.3 is a version that improves overall gameplay with small additions and many code changes which will help me bring my game into multi-player!
The most difficult feature so-far was the grouping of pieces together, so you can stick them together and put them inside the puzzle at once.
Fixing the alignment of pieces for groupings.
My main issue with the grouping was to align them together, at the end the solution is simple since there are few types overall of drag and drop behavior:
- Single piece on single piece
- Group of pieces on a single piece
- Single piece into a group
- Group of pieces on another group of pieces.
But the concern is only on dragging a group and aligning it properly onto another piece.
The algorithm at end was much simpler than I thought, because the other end is static - does not move.
It’s easier to assume the position of the piece that is being dragged and use the difference in location to align the other pieces.
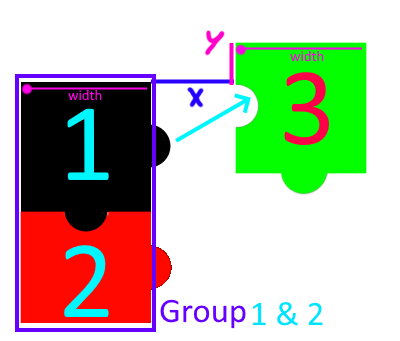
As you can see the X and Y are the difference between the positions and it’s calculated using the width as well.
In games the position is usually calculated as top-left or centered if you use also an Origin point - usually for rotating sprites in their place instead of rotating in arc.
I won’t be getting into right now.
At the end this is how proper alignment looks:
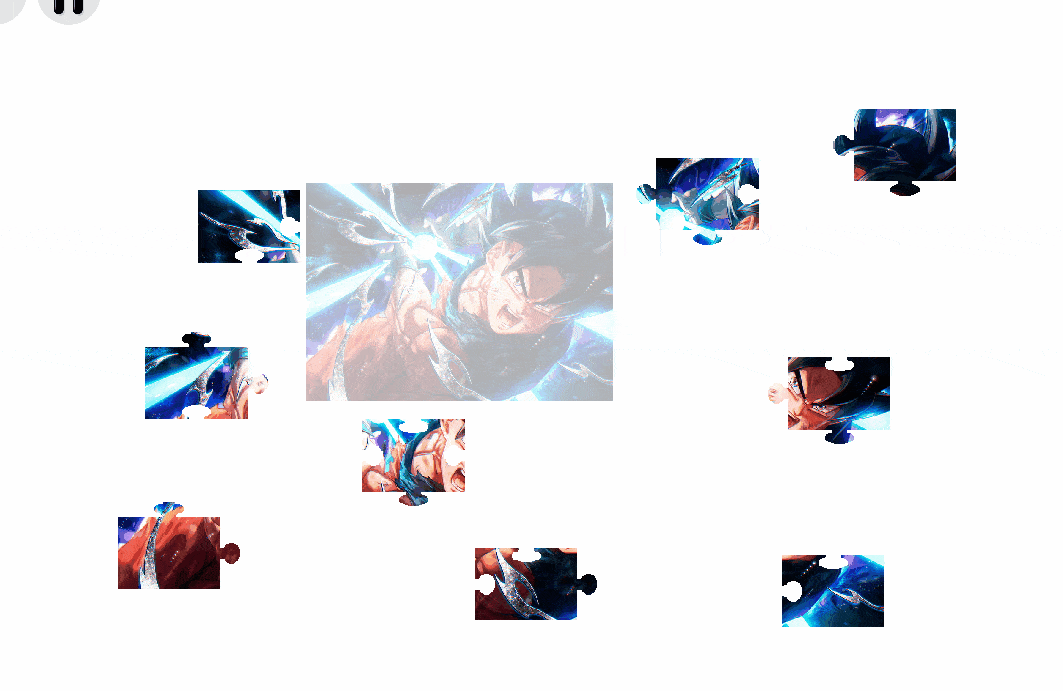
Grouping
Group of pieces is implemented using a class that bonds together pieces.
By this logic when group is aligned with another group I actually merge them together to create a single group!
This way I can control the pieces easily rather than gluing together groups.
The code is actual pretty clever:
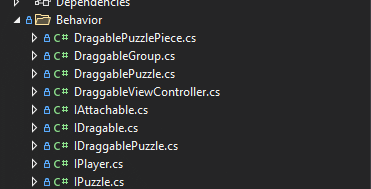
An interface defines a group:
1 | public interface IDraggableGroup<T> : IEnumerable<T> |
Which if you know resembles a List - However I don’t need the whole list behavior so a pre-defined list-like methods are used to explicitly disable the usage of lists.
I actually tried using composition and inheritance however they were all too complicated.
I keep following the rule of KISS - Keep it simple stupid!
And YANGI - Ya ain’t gonna need it!
Because my Draggable pieces are working on events I can use them to sign up to each piece dragging,
Then On dragging I move the position of the other pieces as well.
1 | public void Add(DragablePuzzlePiece draggable) |
This behavior will help me to detect single presses when I join multiple users :)
File selection tree
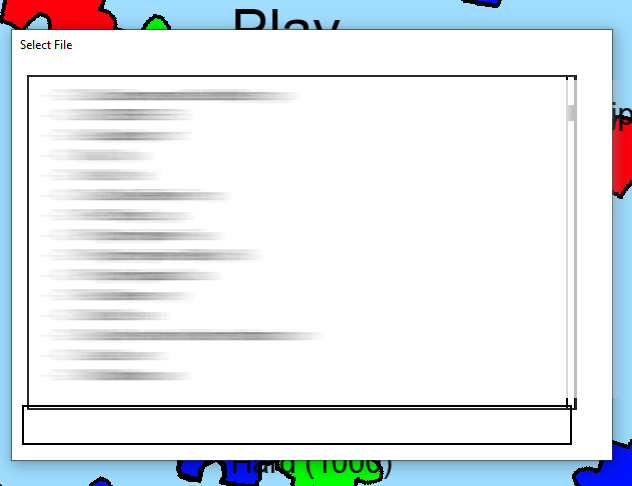
This is exactly as it reads - I re-implement the tree selection for file selection.
This is tedious but kind of fun, rethinking the concepts and implementation details.
I don’t think I actually implemented it very well, I decided to go on a flat list however I think I need to decouple more into:
- Logical and UI components, there are tree nodes, content for the tree nodes (Like file path) and the UI for the tree nodes.
So when I open a folder for example I need to take into account few things: - What logic actually is performed when retreiving children (Or load everything and change the state - more memory I won’t take this approach.).
- How it’s rendered - when opening a folder all UI nodes should move down in the control.
- The coupling between tree node content and UI elements. (Kinda resembles MVVM pattern but I won’t take MVVM fully here).
- Styling - how the element renders.
It needs a lot more work and the graphical refinement will come later!
Road map
The First goal is to produce a functional game which can be played alone or with friends!
The Second goal is to add Multiplayer so people can play together!
The Third goal is to make an enjoyable experience making puzzles - more interactions with the game pieces and games -
- Hints when you are stuck
- Glowing pieces that bring you more points
- Seconds of rush where pieces bring you more points
It’s not easy but very rewarding :)
Hopefully soon I’ll have a #V0.3 to play!